Application Security Project
Application Security Project
Introduction
In this project, my team and I developed a secured API using Python, Flask and SQLite, implementing various security techniques.
​
The context of our project is a sports e-commerce website with an employee portal to manage the inventory, customers and staff in the company. To manage the inventory, customers and staff in the company, the SQLite database was implemented.
​
On my part, I focused on 2 of the OWASP API Top 10 Security Vulnerabilities.
-
Broken User Authentication
-
Broken Function Level Authorization
​​
For each vulnerability, I implemented a number of techniques to mitigate them. To test the implementations, I used the Postman application.
1. Broken User Authentication
-
Password policy​
-
Email confirmation
-
Two-factor authentication
-
Token management
-
Set a maximum number of incorrect login attempts
2. Broken Function Level Authorization
-
​Check logged in user
-
Check staff role
Password policy
The password policy ensures that the password must be at least 8 characters long, and contain the following:
-
at least 1 uppercase letter
-
at least 1 lowercase letter
-
at least 1 numeric character
-
at least 1 special character
Email confirmation
An email is sent out to the newly registered staff to authenticate him/her.
Only after confirming the email will the staff receive a one-time code for the next login.
Two-factor authentication
To implement two-factor authentication, I first used the PyOTP module to generate a one-time code using time-based OTP. Next, I used Twilio to send the generated one-time code to the login user via SMS.
​
For added security, I only made the one-time code valid for 2 minutes after it has been generated. If the one-time code entered for authentication is wrong, the user has to login again to generate a new code.
​
The illustration below shows the use of Postman to verify that the two-factor authentication works.
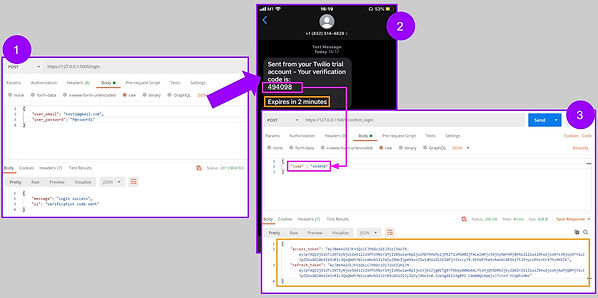
Token management
In this project, JWT tokens were used to validate the logged in user's session. To allow for prolong session use, refresh tokens are created together with the access tokens when the staff logs in to the employee portal.
​
The access token expires after every 5 minutes. When it expires, the refresh token is used to generate a new access token.
​
When the staff logs out of the portal, both the access and refresh tokens are revoked and they can no longer be used for future sessions.
Generation of Access and Refresh Tokens Upon Login
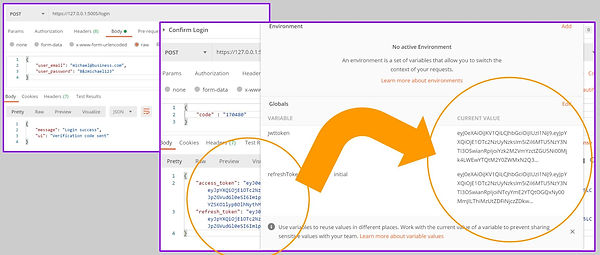
Revocation of Access and Refresh Tokens Upon Logout
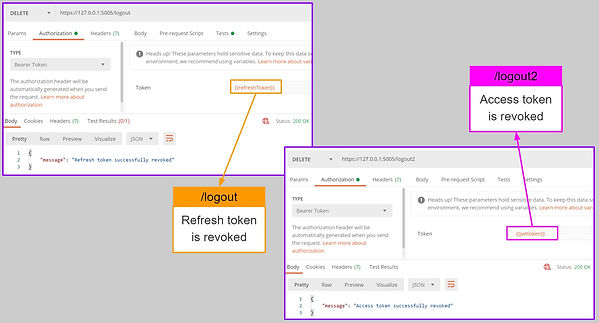
When the user tries to log in again, the old access token is no longer valid.
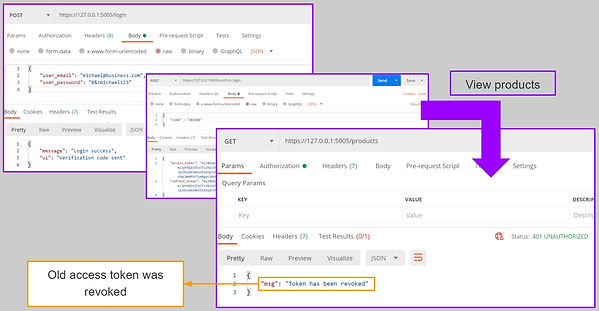
Creation of a New Access Token Using The Refresh Token
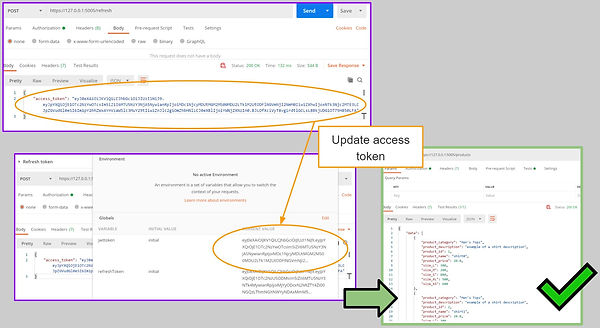
Verifying that the token belongs to the logged-in user
As an added layer of security, in the event the tokens, especially the refresh token, were not properly revoked when the user logs out of the employee portal, I needed to ensure that another user is not able to re-use that token.
​
Hence, I checked the owner of the token using the function get_jwt_identity()
Set a maximum number of incorrect login attempts
To prevent possible brute force attacks from happening, the maximum number of incorrect login attempts is set to 5. Even if the 6th attempt were to be correct, the user will still not be able to gain access to the employee portal.
Check logged in user
To decide on what the logged-in user can and cannot do, I checked the logged-in user object.
If the user is a customer, he/she can only modify his/her own particulars and make purchases.
If the user is a staff, he/she can have permission to manage the inventory, customers, and staff in the company, depending on his/her role.
Check staff role
In the company, there are 3 types of staff. I implemented role-based access control to authorize actions made by the type of staff logged in.
​
The table below is a brief comparison of what each type of staff can manage

Key Takeaways from this Project
I learned about the various OWASP API Top 10 Vulnerabilities and ​techniques that I can use to mitigate them.
​
Starting off with little knowledge on how to implement security in a Flask application, I had to do a lot of research on the python modules and other tools available for me to use.
​
Some interesting python modules that I managed to use were:
-
PyOTP (For generating one-time codes)
-
flask_jwt_extended (For token management)
-
flask_sqlalchemy (For SQLite database)
-
flask_mail (For email confirmation)
-
twilio.rest (For SMS using Twilio)
​